💡 Quotation
SQLite는 MySQL나 PostgreSQL와 같은 데이터베이스 관리 시스템이지만, 서버가 아니라 응용 프로그램에 넣어 사용하는 비교적 가벼운 데이터베이스이다. Wikipedia
게임의 진행 정도, 옵션 등 저장 할 데이터가 있는데 어떤 방식으로 저장을 할까 고민하다가 SQLite 를 사용해 보기로 했습니다. 👍
sqlite3.dll#
SQLite 를 사용하기 위해 두 파일이 필요한데, 먼저 이 곳에 가서 미리 컴파일 된 파일을 다운받고 sqlite3.dll
을 추출합니다.
Mono.Data.Sqlite3.dll#
C:\Program Files\Unity\Hub\Editor\[유니티버전]\Editor\Data\MonoBleedingEdge\lib\mono\4.5
에서 Mono.Data.Sqlite3.dll
파일을 추출합니다.
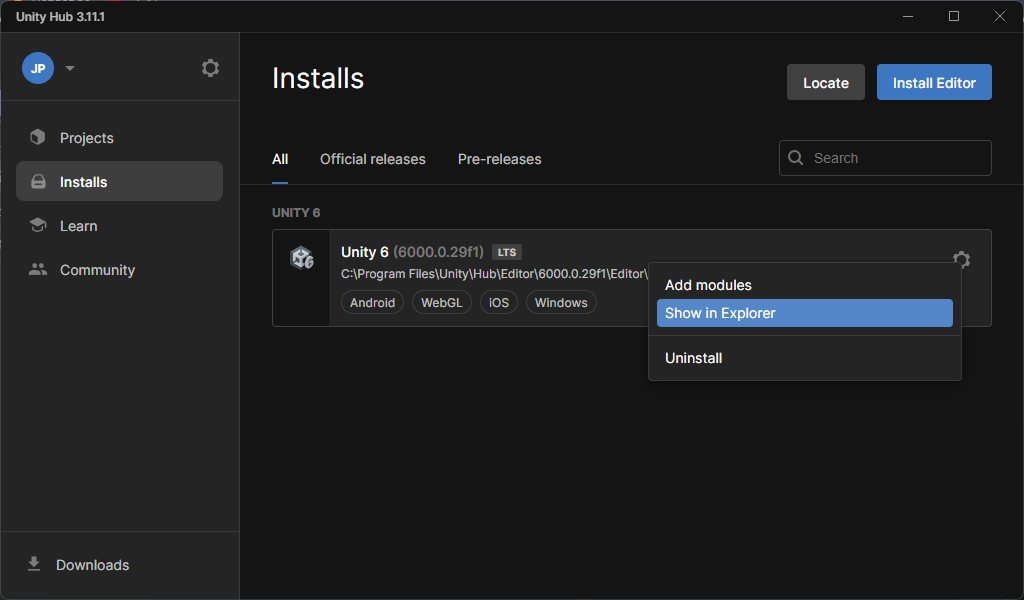
유니티 허브 - Installs 에 설치된 에디터의 톱니바퀴 버튼을 누르면 빠르게 이동할 수 있습니다. 😎
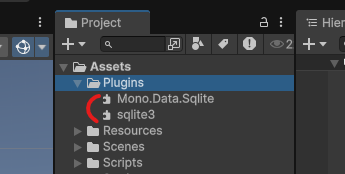
두 파일을 추출했다면 유니티 프로젝트 Assets
폴더에 Plugins
폴더를 만든 후 추가해 주세요.
⚠️ 만약 추가한 후 오류가 생기면 Mono.Data.Sqlite3.dll
을 추출한 곳의 다른 버전을 사용해 보세요.
저는 4.8-api 디렉터리에 있는 파일을 사용했더니 안됐었습니다.
데이터베이스 & 테이블 만들기#
SQLite 의 데이터베이스는 파일로 관리되며 Assets/StreamingAssets/test.db
로 생성했습니다.
만드는 방법은 두 가지 경우가 있습니다.
DBeaver 👍#
🚀 데이터베이스를 만들뿐 아니라 테이블 생성/삭제/조회 등의 쿼리 작업을 위해 사용하는 것을 권장
데이터베이스 최고의 프로그램 DBeaver 가 설치된 경우 “새 연결"을 만들어서 데이터베이스를 바로 생성할 수 있습니다.
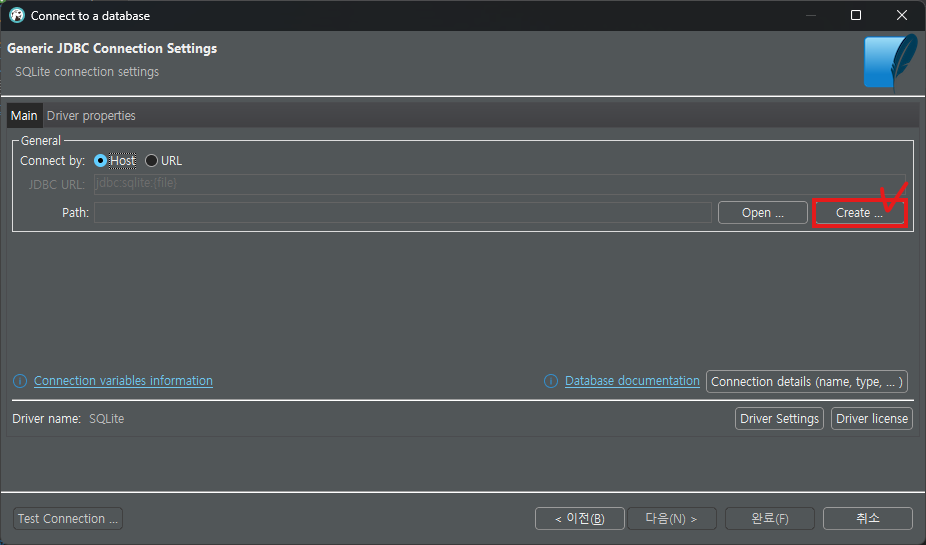
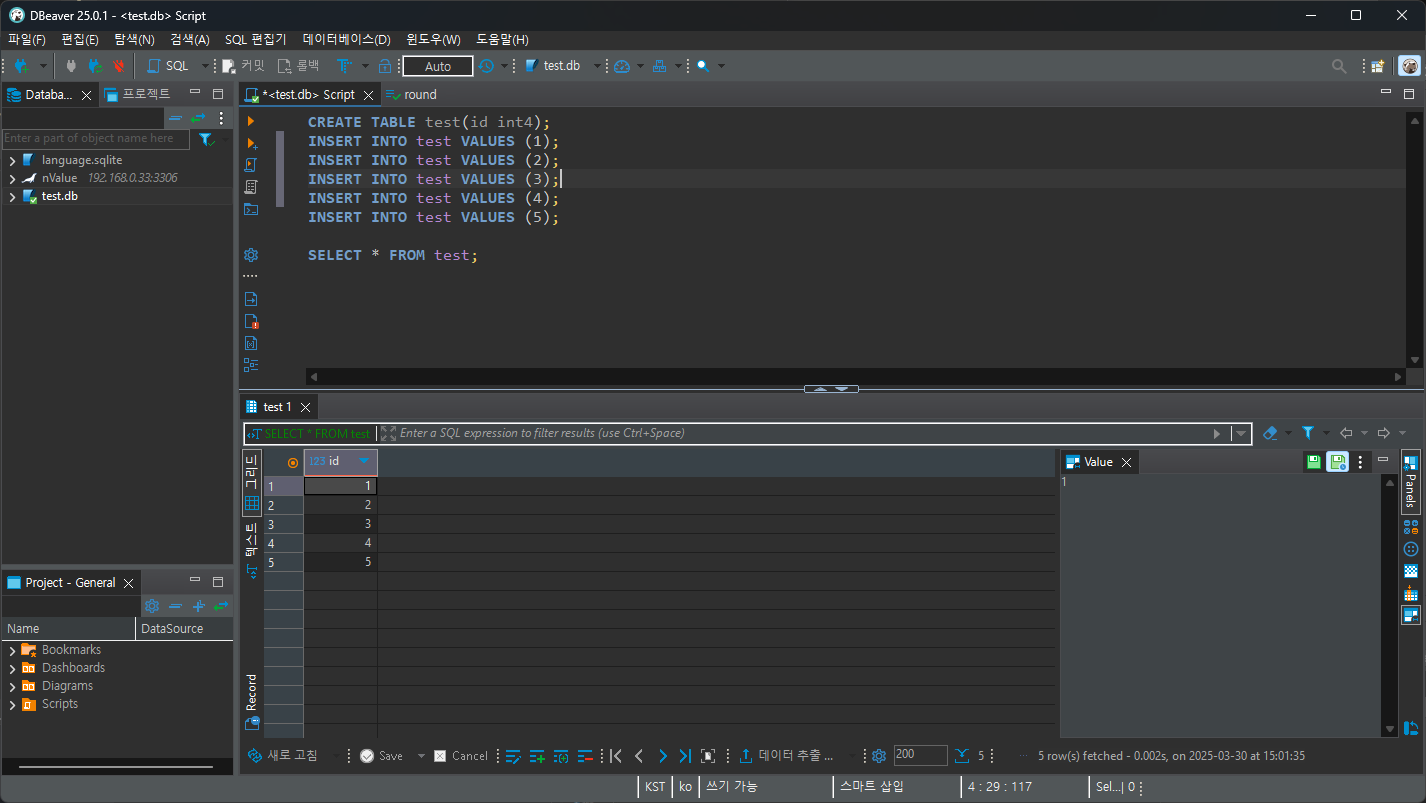
1
2
3
4
5
6
7
8
| CREATE TABLE test(id int4);
INSERT INTO test VALUES (1);
INSERT INTO test VALUES (2);
INSERT INTO test VALUES (3);
INSERT INTO test VALUES (4);
INSERT INTO test VALUES (5);
SELECT * FROM test;
|
sqlite3 CLI#
준비 과정에서 다운 받았던 압축 파일에 sqlite3.exe
를 이용하여 데이터베이스를 생성합니다.
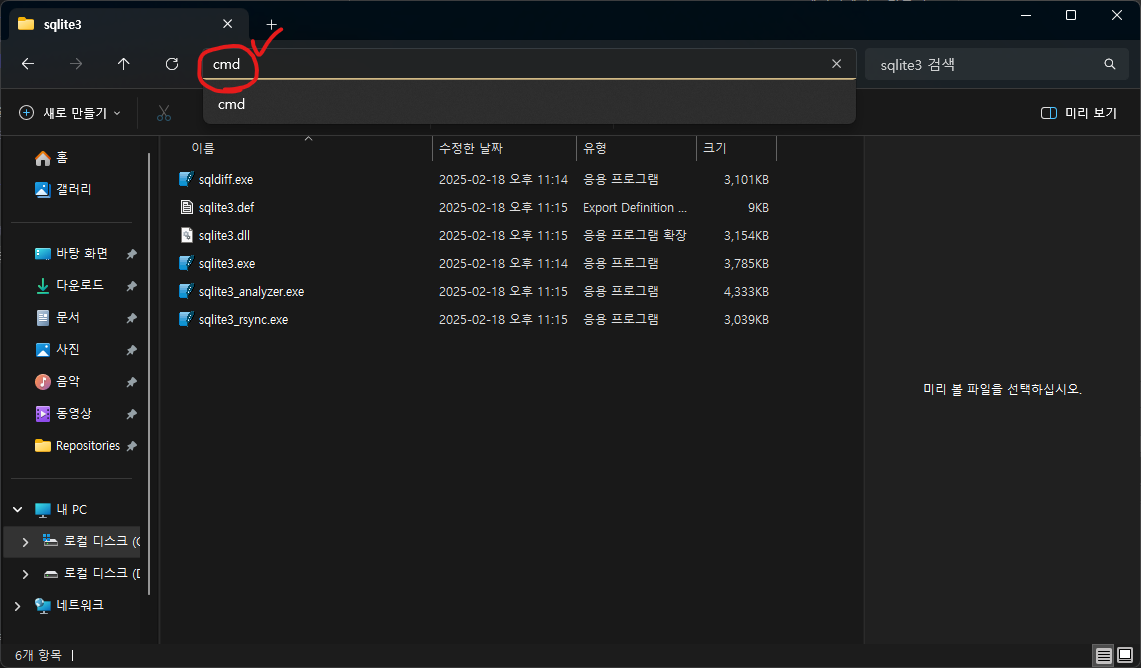
sqlite3.exe
파일이 있는 곳에서 주소표시줄에 cmd
를 입력하고 엔터를 칩니다.
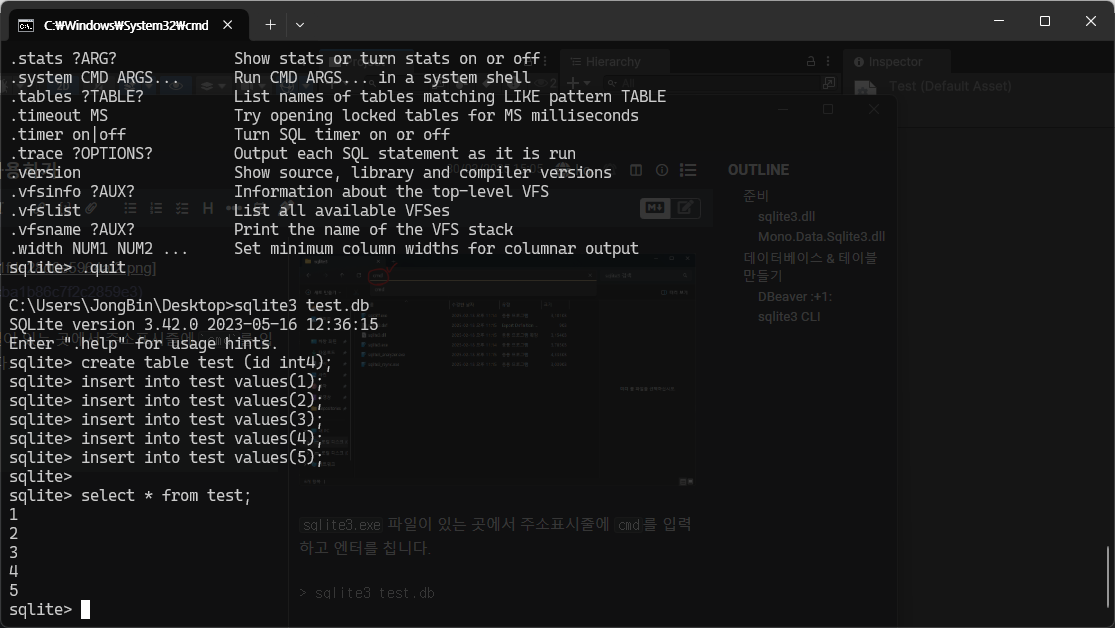
1
2
3
4
5
6
7
8
| CREATE TABLE test(id int4);
INSERT INTO test VALUES (1);
INSERT INTO test VALUES (2);
INSERT INTO test VALUES (3);
INSERT INTO test VALUES (4);
INSERT INTO test VALUES (5);
SELECT * FROM test;
|
test.db 파일을 유니티 프로젝트 폴더에 옮깁니다.
코드 작성#
.NET 에서 제공하는 인터페이스를 사용하여 간단하게 구현 가능합니다.
여기에 Pool 개념을 넣어서 일반화해서 다른 데이터베이스도 같은 메서드로 사용할 수 있게 작성해 줍니다. 😄
Database.cs#
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
| using System;
using System.Collections.Generic;
using System.Data;
using System.Threading;
using System.Threading.Tasks;
public class Database : IDisposable
{
private bool isDestroy;
protected string host;
protected int port;
protected string id;
protected string password;
protected string database;
protected string connectionString;
protected int poolSize;
protected bool isTerminatedWorker;
protected List<Thread> pool;
///////////////////////////////////////////////////////////////////////////////
///
/// critical section
protected object mutex;
protected int connectionCount;
protected Queue<Tuple<string, TaskCompletionSource<IDataReader>>> query;
///
///////////////////////////////////////////////////////////////////////////////
public Database()
{
isDestroy = false;
poolSize = 3;
pool = new List<Thread>();
mutex = new object();
connectionCount = 0;
query = new Queue<Tuple<string, TaskCompletionSource<IDataReader>>>();
}
~Database() { Dispose(false); }
public Database SetHost(string host)
{
this.host = host;
return this;
}
public Database SetPort(int port)
{
this.port = port;
return this;
}
public Database SetId(string id)
{
this.id = id;
return this;
}
public Database SetPassword(string password)
{
this.password = password;
return this;
}
public Database SetDatabase(string database)
{
this.database = database;
return this;
}
public Database SetPoolSize(int size)
{
this.poolSize = size;
return this;
}
public virtual void Open() { }
public void Close()
{
isTerminatedWorker = true;
lock (mutex)
Monitor.PulseAll(mutex);
foreach (Thread thread in pool)
thread.Join();
pool.Clear();
}
public int GetConnectionCount() => connectionCount;
public int GetPoolSize() => poolSize;
public string GetConnectionString() => connectionString;
public Task<IDataReader> Execute(string queryString)
{
TaskCompletionSource<IDataReader> result = new TaskCompletionSource<IDataReader>();
Tuple<string, TaskCompletionSource<IDataReader>> job = Tuple.Create(queryString, result);
lock (mutex)
{
query.Enqueue(job);
Monitor.Pulse(mutex);
}
return result.Task;
}
public void Dispose()
{
Dispose(true);
GC.SuppressFinalize(this);
}
protected virtual void Dispose(bool disposing)
{
if (isDestroy)
return;
if (disposing)
{
}
Close();
isDestroy = true;
}
protected void Worker(IDbConnection connection)
{
Tuple<string, TaskCompletionSource<IDataReader>> job;
connection.Open();
if (connection.State == ConnectionState.Open)
lock (mutex)
connectionCount++;
while (!isTerminatedWorker)
{
lock(mutex)
{
if (query.Count == 0)
Monitor.Wait(mutex);
if (query.Count == 0)
continue;
if (isTerminatedWorker && query.Count == 0)
break;
job = query.Dequeue();
}
using (IDbCommand command = connection.CreateCommand())
{
command.CommandText = job.Item1;
job.Item2.SetResult(command.ExecuteReader());
}
}
connection.Close();
if (connection.State == ConnectionState.Closed)
lock (mutex)
connectionCount--;
connection.Dispose();
}
}
|
SQLite.cs#
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
| using System.Data;
using Mono.Data.Sqlite;
using UnityEngine;
using System.Threading;
public class SQLite : Database
{
public override void Open()
{
isTerminatedWorker = false;
connectionString = "URI=file:" + Application.streamingAssetsPath + "/" + database;
int _currentConnection = connectionCount;
for (int i = 0; i < poolSize - _currentConnection; i++)
{
IDbConnection conn = new SqliteConnection(connectionString);
Thread thread = new Thread(() => { Worker(conn); });
thread.Start();
pool.Add(thread);
}
}
}
|
단위 테스트 코드 작성#
SQLiteTest.cs#
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
| using System.Collections;
using System.Data;
using System.Threading;
using System.Threading.Tasks;
using NUnit.Framework;
using UnityEngine;
using UnityEngine.TestTools;
public class SQLiteTest
{
[Test]
public void OpenTestPasses()
{
SQLite db = new SQLite();
db.SetDatabase("test.db");
db.Open();
Thread.Sleep(100); // 활성화 시간
Assert.AreEqual(db.GetPoolSize(), db.GetConnectionCount());
}
[Test]
public void CloseTestPasses()
{
SQLite db = new SQLite();
db.SetDatabase("test.db");
db.Open();
Thread.Sleep(100); // 활성화 시간
db.Close();
Thread.Sleep(100); // 활성화 시간
Assert.AreEqual(db.GetConnectionCount(), 0);
}
[Test]
public void DuplicateOpenTestPasses()
{
SQLite db = new SQLite();
db.SetDatabase("test.db");
db.Open();
Thread.Sleep(100); // 활성화 시간
Assert.AreEqual(db.GetPoolSize(), db.GetConnectionCount());
db.Open();
Thread.Sleep(100); // 활성화 시간
Assert.AreEqual(db.GetPoolSize(), db.GetConnectionCount());
db.Open();
Thread.Sleep(100); // 활성화 시간
Assert.AreEqual(db.GetPoolSize(), db.GetConnectionCount());
db.Open();
Thread.Sleep(100); // 활성화 시간
Assert.AreEqual(db.GetPoolSize(), db.GetConnectionCount());
}
[Test]
public void ExecuteTestPasses()
{
SQLite db = new SQLite();
db.SetDatabase("test.db");
db.Open();
int i = 1;
using (IDataReader reader = db.Execute("select * from test").Result)
{
while (reader.Read())
{
Assert.AreEqual(i++, reader.GetInt32(0));
}
}
}
[Test]
public void ExecuteDirtyTestPasses()
{
int tryCount = 10000;
SQLite db = new SQLite();
db.SetDatabase("test.db");
db.Open();
int i;
for (int t=0; t < tryCount; t++)
{
i = 1;
using (IDataReader reader = db.Execute("select * from test").Result)
{
while (reader.Read())
{
Assert.AreEqual(i++, reader.GetInt32(0));
}
}
}
}
}
|
테스트#
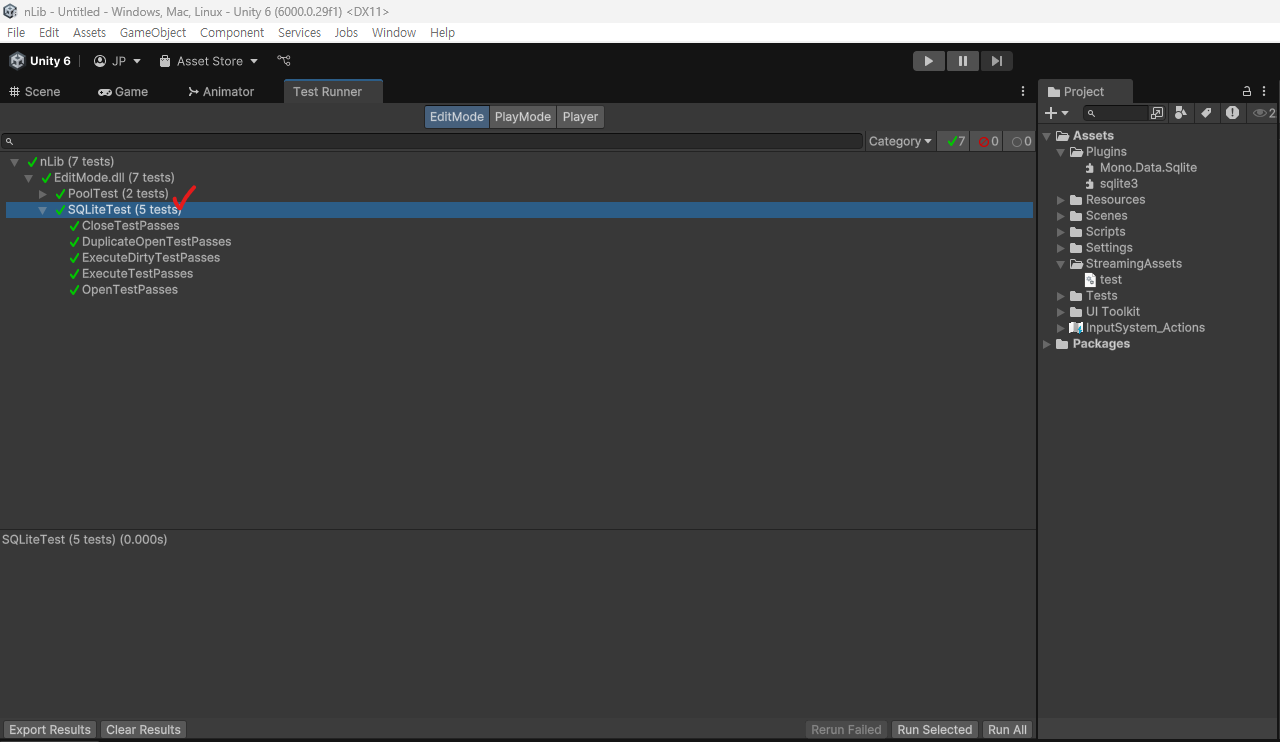
- 연결
- 연결해제
- 중복연결
- 테이블 조회 1번
- 테이블 조회 1만번